Python: Learn Python in 1 Hour
This is a Python tutorial. Spend 1 hour, and you will have a basic understanding of the language.
Examples on this page are based on Python 2.7. (python 2.x is no longer supported since year 2020)
For python 3, see: Python 3 Tutorial .
Run Python Program
create a file with this content:
# python 2 print 1+2
Save the file as “test.py”.
To run it, go to terminal, type
python test.py
Python will print the output 3.
Or, you can run short python expressions in a command line interface. Go to terminal, type “python” to start.
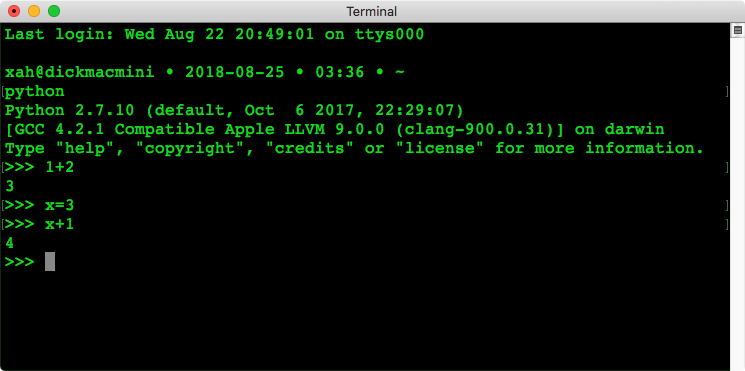
To exit, press Ctrl+d on a new line.
Printing
# -*- coding: utf-8 -*- # python 2 print 3 print 3, 4
In python 2, print
is a statement. You do not need parenthesis after it. It can have multiple values, separated by comma.
Strings
Strings are enclosed using 'single' quote or "double" quote.
# -*- coding: utf-8 -*- # python 2 b = 'rabbit' # single quotes a = "tiger" # double quotes print a, b # tiger rabbit
You can use \n
for linebreak, and \t
for tab.
Single quote and double quote syntax have the same meaning.
Quoting Raw String r"…"
Add r
in front of the string quote symbol for raw string. This way, backslash characters will NOT be interpreted as escapes. (“r” for “raw”)
c = r"this\n and that" print c # prints a single line
Triple Quotes for Multi-Line String
To quote a string of multiple lines, use triple quotes like this '''…'''
or """…"""
.
d = """this will be printed in 3 lines""" print d
For detail, see: Python: Quote String
Unicode in String or Source File
If anywhere in your source code file contains Unicode characters, the first or second line should be:
# -*- coding: utf-8 -*-
Any string containing Unicode characters should have “u” prefix, for example, u"i ♥ cats"
.
# -*- coding: utf-8 -*- # python 2 a = u"I ♥ cats" # string with unicode heart ♥
For detail, see: Python: Unicode Tutorial 🐍 .
Substring
string[begin_index:end_index]
- Return a substring of string with index begin_index to end_index.
- Index starts at 0.
- The returned string does not include end_index.
- Index can be negative, which counts from the end.
# -*- coding: utf-8 -*- # python 2 print "01234567"[1:4] # 123
# -*- coding: utf-8 -*- # python 2 b="01234567" print b[1:4] # 123 print b[1:-1] # 123456 print b[-2:-1] # 6
String Length
len(str)
- Return the number of chars in is string str.
print len("abc") # 3
String Join
Join string: string + string
.
print "abc" + " xyz" # "abc xyz"
String Repeat
String can be repeated using *
.
print "ab" * 3 # "ababab"
String Methods
Arithmetic
# -*- coding: utf-8 -*- # python 2 print 3 + 4 # 7 print 3 - 4 # -1 print 3 + - 4 # -1 print 3 * 4 # 12
Division, Quotient, Remainder (mod)
# -*- coding: utf-8 -*- # python 2 # quotient # dividing two integers is integer print 11 / 5 # 2 # quotient with a float number print 11 / 5. # 2.2 # integer part of quotient print 11 // 5 # 2 print 11 // 5. # 2.0 # remainder, modulo print 11 % 5 # 1 print 11 % 5. # 1.0 # quotient and remainder print divmod(11, 5) # (2, 1) # quotient and remainder print divmod(11, 5.) # (2.0, 1.0)
Warning: in Python 2, 11/5
returns 2, not 2.2. Use float 11/5.
.
Power, Root
# -*- coding: utf-8 -*- # python 2 # power print 2 ** 3 # 8 # square root print 8 **(1/3.) # 2.0
In Python, power is **
. The ^
is used for bitwise xor. [see Python: Operators]
Convert to {int, float, string}
Python doesn't automatically convert between {int, float, string}.
- Convert to int:
int(3.2)
. - Convert to float:
float(3)
. - Convert to string, use
repr(123)
orstring.format(…)
method. For example,"integer {:d}, float {:f}".format(3, 3.2)
. [see Python: Format String] - You can write with a dot after the number as float, like this:
3.
.
Assignment
# -*- coding: utf-8 -*- # python 2 # add and assign c = 0 c += 1 print c # 1 # substract and assign c = 0 c -= 2 print c # -2 # multiply and assign c = 2 c *= 3 print c # 6 # power and assign c = 3 c **= 2 print c # 9 # divide and assign c = 7 c /= 2 print c # 3 Note: not 3.5 # modulus (remainder) and assign c = 13 c %= 5 print c # 3 # quotient and assign c = 13 c //= 5 print c # 2
Note: Python doesn't support ++
or --
.
Warning: ++i
may not generate any error, but it doesn't do anything.
For bitwise and other operators, see: Python: Operators .
True and False
True
and non-empty things are true. Number1
is true.False
,None
, zero, and empty things are false.
# -*- coding: utf-8 -*- # python 2 my_thing = [] if my_thing: print "yes" else: print "no" # prints no
[see Python: True, False (boolean)]
Conditional: if then else
# -*- coding: utf-8 -*- # python 2 x = -1 if x < 0: print 'neg'
# -*- coding: utf-8 -*- # python 2 x = -1 if x < 0: print 'negative' else: print '0 or positive'
# -*- coding: utf-8 -*- # python 2 # Examples of if x = -1 if x<0: print 'neg' elif x==0: print 'zero' elif x==1: print 'one' else: print 'other' # the elif can be omitted.
Loop, Iteration
while loop.
# -*- coding: utf-8 -*- # python 2 x = 1 while x < 9: print x x += 1
for loop.
# -*- coding: utf-8 -*- # python 2 # creates a list from 1 to 3. (does NOT include 4) a = range(1,4) for x in a: print x
The range(m, n)
function gives a list from m to n, not including n.
Python also supports break
and continue
to exit loop.
break
- Exit loop.
continue
- Skip code and start the next iteration.
# -*- coding: utf-8 -*- # python 2 for x in range(1,9): print 'yay:', x if x == 5: break
List
Creating a list.
a = [0, 1, 2, "more", 4, 5, 6] print a
Counting elements:
a = ["more", 4, 6] print len(a) # prints 3
Getting a element. Use the syntax list[index]
. Index start at 0. Negative index counts from right. Last element has index -1.
a = ["more", 4, 6] print a[1] # prints 4
Extracting a sequence of elements (aka sublist, slice): list[start_index:end_index]
.
# -*- coding: utf-8 -*- a = [ "b0", "b1", "b2", "b3", "b4", "b5", "b6"] print a[2:4] # → ['b2', 'b3']
WARNING: The extraction does not include the element at the end index. For example, myList[2:4]
returns only 2 elements, not 3.
Modify element: list[index] = newValue
# -*- coding: utf-8 -*- a = ["b0", "b1", "b2"] a[2] = "two" print a # → ['b0', 'b1', 'two']
A slice (continuous sequence) of elements can be changed by assigning to a list directly. The length of the slice need not match the length of new list.
# -*- coding: utf-8 -*- # python 2 xx = [ "b0", "b1", "b2", "b3", "b4", "b5", "b6"] xx[0:6] = ["two", "three"] print xx # ['two', 'three', 'b6']
Nested Lists. Lists can be nested arbitrarily. Append extra bracket to get element of nested list.
# -*- coding: utf-8 -*- bb = [3, 4, [7, 8]] print bb # [3, 4, [7, 8]] print bb[2][1] # 8
List Join. Lists can be joined with plus sign.
b = ["a", "b"] + [7, 6] print b # prints ['a', 'b', 7, 6]
Python Data Structure
- Python: List
- Python: Generate List: range
- Python: Generate List (List Comprehension)
- Python: List Methods
- Python: Loop Thru List
- Python: Map, Filter, List
- Python: Iterator to List
- Python: Copy Nested List, Shallow/Deep Copy
- Python: Sort
- Python: Dictionary
- Python: Loop Thru Dictionary
- Python: Dictionary Methods
- Python: Tuple
- Python: Sets, Union, Intersection
- Python: Sequence Types
- Python: Read/Write to JSON
Tuple
Python has a “tuple” type. It's like list, except that the elements cannot be changed, nor can new element added.
Syntax for tuble is using round brackets () instead of square brackets. The brackets are optional when not ambiguous, but best to always use them.
# -*- coding: utf-8 -*- # python 2 # tuple t1 = (3, 4 , 5) # a tuple of 3 elements print t1 # (3, 4 , 5) print t1[0] # 3 # nested tuple t2 = ((3,8), (4,9), ("a", 5, 5)) print t2[0] # (3,8) print t2[0][0] # 3 # a list of tuples t3 = [(3,8), (4,9), (2,1)] print t3[0] # (3,8) print t3[0][0] # 3
[see Python: Tuple]
Python Sequence Types
In Python, {string, list, tuple} are called “sequence types”. Here's example of operations that can be used on sequence type.
# length ss = [0, 1, 2, 3, 4, 5, 6] print len(ss) # 7
# ith item ss = [0, 1, 2, 3, 4, 5, 6] print ss[0] # 0
# slice of items ss = [0, 1, 2, 3, 4, 5, 6] print ss[0:3] # [0, 1, 2]
# slice of items with jump step ss = [0, 1, 2, 3, 4, 5, 6] print ss[0:10:2] # [0, 2, 4, 6]
# check if a element exist ss = [0, 1, 2, 3, 4, 5, 6] print 3 in ss # True. (or False)
# check if a element does NOT exist ss = [0, 1, 2, 3, 4, 5, 6] print 3 not in ss # False
# concatenation ss = [0, 1] print ss + ss # [0, 1, 0, 1]
# repeat ss = [0, 1] print ss * 2 # [0, 1, 0, 1]
# smallest item ss = [0, 1, 2, 3, 4, 5, 6] print min(ss) # 0
# largest item ss = [0, 1, 2, 3, 4, 5, 6] print max(ss) # 6
# index of the first occurrence ss = [0, 1, 2, 3, 4, 5, 6] print ss.index(3) # 3
# total number of occurrences ss = [0, 1, 2, 3, 4, 5, 6] print ss.count(3) # 1
Python Data Structure
- Python: List
- Python: Generate List: range
- Python: Generate List (List Comprehension)
- Python: List Methods
- Python: Loop Thru List
- Python: Map, Filter, List
- Python: Iterator to List
- Python: Copy Nested List, Shallow/Deep Copy
- Python: Sort
- Python: Dictionary
- Python: Loop Thru Dictionary
- Python: Dictionary Methods
- Python: Tuple
- Python: Sets, Union, Intersection
- Python: Sequence Types
- Python: Read/Write to JSON
Dictionary: Key/Value Pairs
A keyed list in Python is called “dictionary” (known as Hash Table or Associative List in other languages). It is a unordered list of pairs, each pair is a key and a value.
# -*- coding: utf-8 -*- # python 2 # define a keyed list aa = {"john":3, "mary":4, "joe":5, "vicky":7} print "aa is:", aa # getting value from a key print "mary is:", aa["mary"] # add a entry aa["pretty"] = 99 print "added pretty:", aa # delete a entry del aa["vicky"] print "deleted vicky", aa # get just the keys print "just keys", aa.keys() # to get just values, use “.values()” # check if a key exists print "is mary there:", aa.has_key("mary")
Python Data Structure
- Python: List
- Python: Generate List: range
- Python: Generate List (List Comprehension)
- Python: List Methods
- Python: Loop Thru List
- Python: Map, Filter, List
- Python: Iterator to List
- Python: Copy Nested List, Shallow/Deep Copy
- Python: Sort
- Python: Dictionary
- Python: Loop Thru Dictionary
- Python: Dictionary Methods
- Python: Tuple
- Python: Sets, Union, Intersection
- Python: Sequence Types
- Python: Read/Write to JSON
Enumerate List/Dictionary
Here is a example of going thru a list by element.
myList=['one', 'two', 'three', 'infinity'] for x in myList: print x
You can loop thru a list and get both {index, value} of a element.
myList=['one', 'two', 'three', 'infinity'] for i, v in enumerate(myList): print i, v # 0 one # 1 two # 2 three # 3 infinity
The following construct loops thru a dictionary, each time assigning both keys and values to variables.
myDict = {'john':3, 'mary':4, 'joe':5, 'vicky':7} for k, v in myDict.iteritems(): print k, ' is ', v
[see Python: Map, Filter, List]
Module and Package
A library in Python is called a module. A collection of module is called a package.
To load a module, call import moduleName
. Then, to use a function in the module, use moduleName.functionName(…)
.
# -*- coding: utf-8 -*- # python 2 # import the standard module named os import os # example of using a function print 'current dir is:', os.getcwd()
# -*- coding: utf-8 -*- # python 2 import os # print all names exported by the module print dir(os)
[see Python: List Modules, List Loaded Modules]
Defining a Function
The following is a example of defining a function.
def myFun(x,y): """myFun returns x+y.""" result = x+y return result print myFun(3,4) # 7
The string immediately following the first line is the function's documentation.
A function can have named optional parameters. If no argument is given, a default value is assumed.
def myFun(x, y=1): """myFun returns x+y. Parameter y is optional and default to 1""" return x+y print myFun(3,7) # 10 print myFun(3) # 4
[see Python: Function]
Class and Object
Writing a Module
Here's a basic example. Save the following line in a file and name it mm.py
.
def f3(n): return n+1
To load the file, use import import mm
. To call the function, use mm.f3
.
import mm # import the module print mm.f3(5) # calling its function. prints 6 print mm.__name__ # list its functions and variables