Official Java Tutorial on Interface, the Inanity
In English, an interface is a device or a system that unrelated entities use to interact. According to this definition, a remote control is an interface between you and a television set, the English language is an interface between two people, and the protocol of behavior enforced in the military is the interface between people of different ranks. Within the Java programming language, an interface is a device that unrelated objects use to interact with each other. An interface is probably most analogous to a protocol (an agreed on behavior). In fact, other object-oriented languages have the functionality of interfaces, but they call their interfaces protocols.
—Official Java Tutorial,
The Concept of Interface
In most languages, a function/subroutine can be specified by its name and parameter specs. For example:
f(3)
f(3, [9,2])
f("some string")
Above are examples of 3 function calls. The functions all having the same name, but have different number and type of arguments.
A function is essentially known to outsiders by its name and parameter specs. The gist in this concept is that the user don't need to know the implementation details of the function. All you need to know is the function's name, and parameter specs and return value spec. (and of course what the function is supposed to do.) In this way, interface and implementation are separated. The implementation can change or improve anytime, and you don't need to know about it in order to use it.
In Java, the above concept of function name and parameter spec is called a method's “signature”.
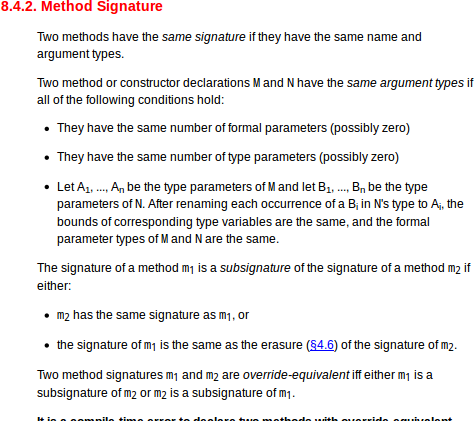
For another example, usually a program needs to talk to another software such as a database software. The database software may have a set of functions for the purpose of communicating to other software. In essence, making the database useful to other software. Such a list of function spec is often called API, which stands for Application Programing Interface.
The API terminology is abused by the marketing-loving Sun Microsystems by calling the Java language's documentation as “The Java API”, even though Java the language and its paraphernalia of libraries and hardware-emulation system (all together jargonized as the Java “Platform”) isn't a Application nor Interface. (a API implies that there are two disparate entities involved, which are allowed to communicate thru it. (For example, Google Map API, ImageMagick's API, Database API, credit card network API) ) In the case of “The Java API”, it's one entity talking to itself.
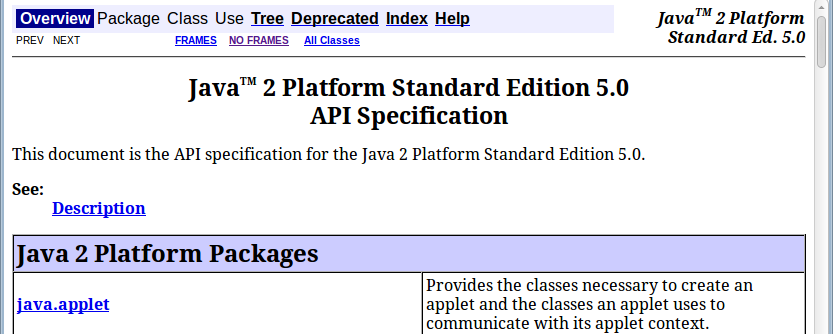
In general, the interface concept in programing is a sort of specification that allows different entities to call and make use of the other, with the implication that the caller need not know what's behind the facade.
In the Object Oriented Programing Paradigm, a new concept arose, that is the “interface” aspect of a class.
As we've seen, a function has parameter spec that is all there it is a user needs to know for using it. In Java, this is the method's “signature”. Now, as the methodology of the OOP experience multiplies, it became apparent that the interface concept can be applied to Classes as well. Specifically: the interface of a class is the class's methods.
This concept is then turned into a OOP machinery, in hope of extracting usefulness in software engineering. That is to say, now in the Java language, a programer can actually write a piece of code, whose sole purpose is to define what methods and variables a class contains. This, is done with the keyword interface
. Once a interface is defined, other classes can say which interfaces they implement, so that if class C implement interface I, then programers don't need to know the details about C. All they need to know is the interface I. (which specifies all the methods, constructors, variables, a class must have.)
(Java's interface, is essentially the “signature” of a class, in Sun Microsystem's own jargon.)
Interface in Java
A programer may ask, what's the big deal anyway? Since in Java, classes are well documented anyway. What difference does it make to know the documentation of C versus the documentation of interface for C?
Interfaces Can Have Hierarchical Relations
The thing about interface in Java is that the complexity grows. A Java interface, can be inherited, just as classes. The idea is that interfaces can also form a hierarchy just like classes.
In OOP language such as Java, the object entities used to solve computing problems are thought to form a relation as of a tree, thus we have the class hierarchy. In a similar way, it is thought that interface, can also form a hierarchy fruitfully. A good example is the list data type. The explanation follows.
In computing languages, often there's a data concept variously known as list, aggregate, sequence, array, vector, tuple, set, matrix, tree. The basic idea is that it just a collection of things treated as one single entity. This collective may not allow repetitions (a “set”), elements may be nested (tree), may have certain structure constrains when nested (matrix), may have certain computational properties such as speed of retrieving a element or adding a element or memory footprint (vector, array, hash table, associative list) etc and so on. Different requirement and different computational properties have given them various names to go by. One can however organize them by the interface perspective. In Java, they are known as Collection, and all have the interface of Collection.
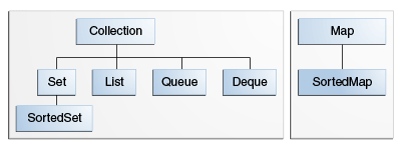
[Collection (Java Platform SE 8 ) ../java8_doc/api/java/util/Collection.html]
Consider a Set and List. Their difference is that Set does not allow repeated element, while List does. And, Set are typically thought of as without a order, while List often does. Other than that, both concepts are the same. They both need methods like adding elements, deleting, inserting, etc. Therefore, from interface point of view, they share a parent. In Java, both Set and List are interfaces, inherited from the parent interface Collection.
A Class Can Have Multiple Interfaces
When a class C has interface I, it is said to “implement” that interface.
In Java, it's so designed that a class can implement more than one interfaces (or none). When a class C implements interfaces I1 and I2, C is guaranteed to have all methods declared by interface I1 and I2. For example, in Java, class Integer has interfaces Comparable and Serializable. And the class ArrayList has these interfaces: {Cloneable, Collection, List, RandomAccess, Serializable}.
For example, see the Java documentation on these interfaces:
- [ArrayList (Java Platform SE 8 ) ../java8_doc/api/java/util/ArrayList.html]
- [RandomAccess (Java Platform SE 8 ) ../java8_doc/api/java/util/RandomAccess.html]
- [Serializable (Java Platform SE 8 ) ../java8_doc/api/java/io/Serializable.html]
So now, in Java, we have two hierarchies of separate category: Classes and Interfaces. The Classes hierarchy is one single giant tree. However, the interfaces are not all together as one tree. They are more like forests, of many trees. That is, many separate group of hierarchy. It is important to remember that interfaces and classes are separate entities.
Interface as Label: Marker Interface
It is curious, that if you look at some of the interfaces, such as RandomAccess and Serializable, they do not declare a single method or variable. What good is a interface when it doesn't have a single member?
For example, the ArrayList class has these interfaces: {Cloneable, RandomAccess, Serializable}. None of these interfaces declares a single member. As one can infer from the names, they seem to suggest some computational properties.
One might ask, in “interfaces” such as RandomAccess that doesn't have a single variable or method, in what technical definition that a class is said to satisfy such interfaces? And, given the existence of these property-like interfaces, can a programer define their own arbitrary computational property contract? For example, suppose i want a property ConstantTime for a class in a game i'm developing. Once i declared a class to have “interface” ConstantTime, apparently my class is not going to magically become constant time. How do i define arbitrary properties to the compiler, and how's the compiler going to verify it?
Java's Interface has mutated so much from the interface concept that it also functions as a pure label. If a interface does not have any variables or methods, any class can declare it as a interface. There is no restraint whatsoever. For example, the RandomAccess interface in Java does not have any variables or methods. Any class can declare it as a interface, randomly accessible or not. When interface is used as a label, it is called a marker interface by the Java documentation. For example, see [RandomAccess (Java Platform SE 8 ) java8_doc/api/java/util/RandomAccess.html]
As a pure label, a programer can create “marker interfaces” such as {blue, red, yellow} to attach to classes, as well as “JohnsJob”, “Experimental”.
Because the multi-inheritance nature of Java interface, and its double role as a label, it no longer function as a communication facade that is the normal meaning of the word interface. If a Java class have interfaces {A, B, C, D, E}, then you cannot be sure just exactly what methods or variables the class have. (it will be a union of them, and some of them do not serve any technical function in language other than as a label to the programer to help in writing programs)
Using interface as a inert label to indicate computational properties (For example, RandomAccess) is a egregious incompetence in the design of a language for computation. The gist of the problem is that it is a piece of mathematical irrelevance in the language. As a labeling mechanism in a language, for the possible benefit from the software engineering perspective, then it should not be designed as part of the Class Interface, since the concept of labeling, and concept of programing interface, are semantically disparate.
The Inanity of Java Tutorials
The Java tutorials out there are often inane, in that none of them actually tried to teach what the language actually manifestly do, but instead, often talk in some purportedly good engineering perspective.
For a incomprehensible babble of interface in English see this official Java tutorial of 2005:
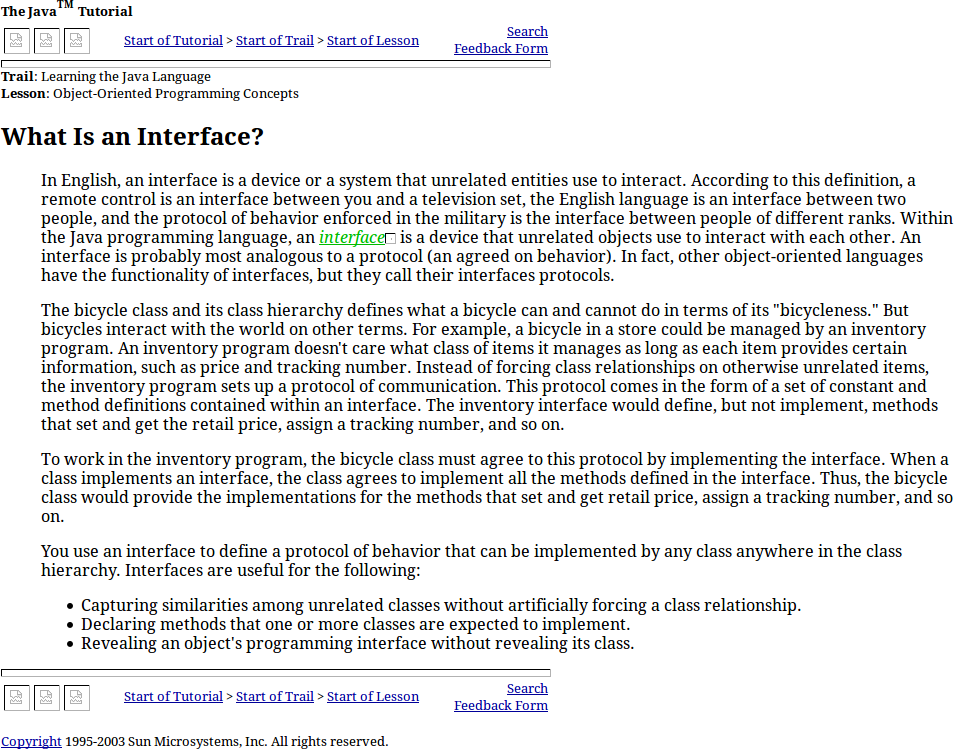
For a incomprehensible metaphysical babble of interface with bicycle , see this official Java tutorial of 2011:
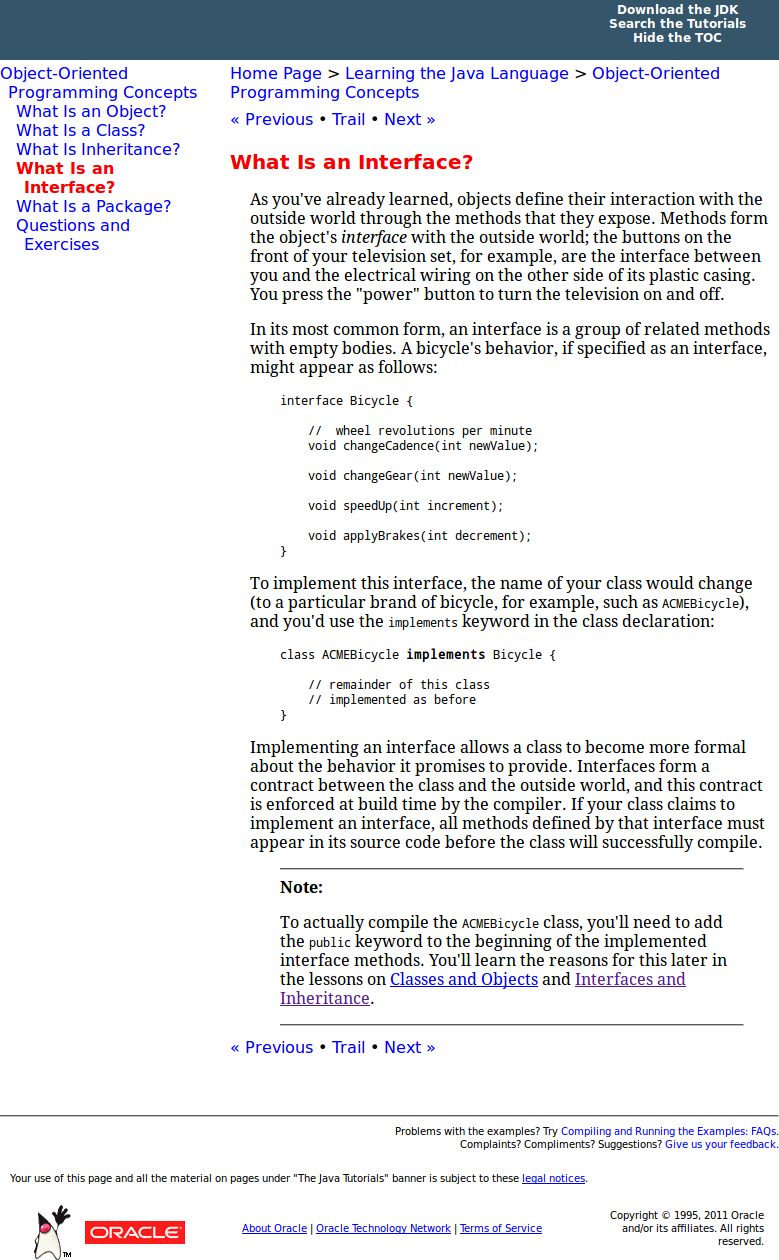
For a show of baffling financial stocks, see:
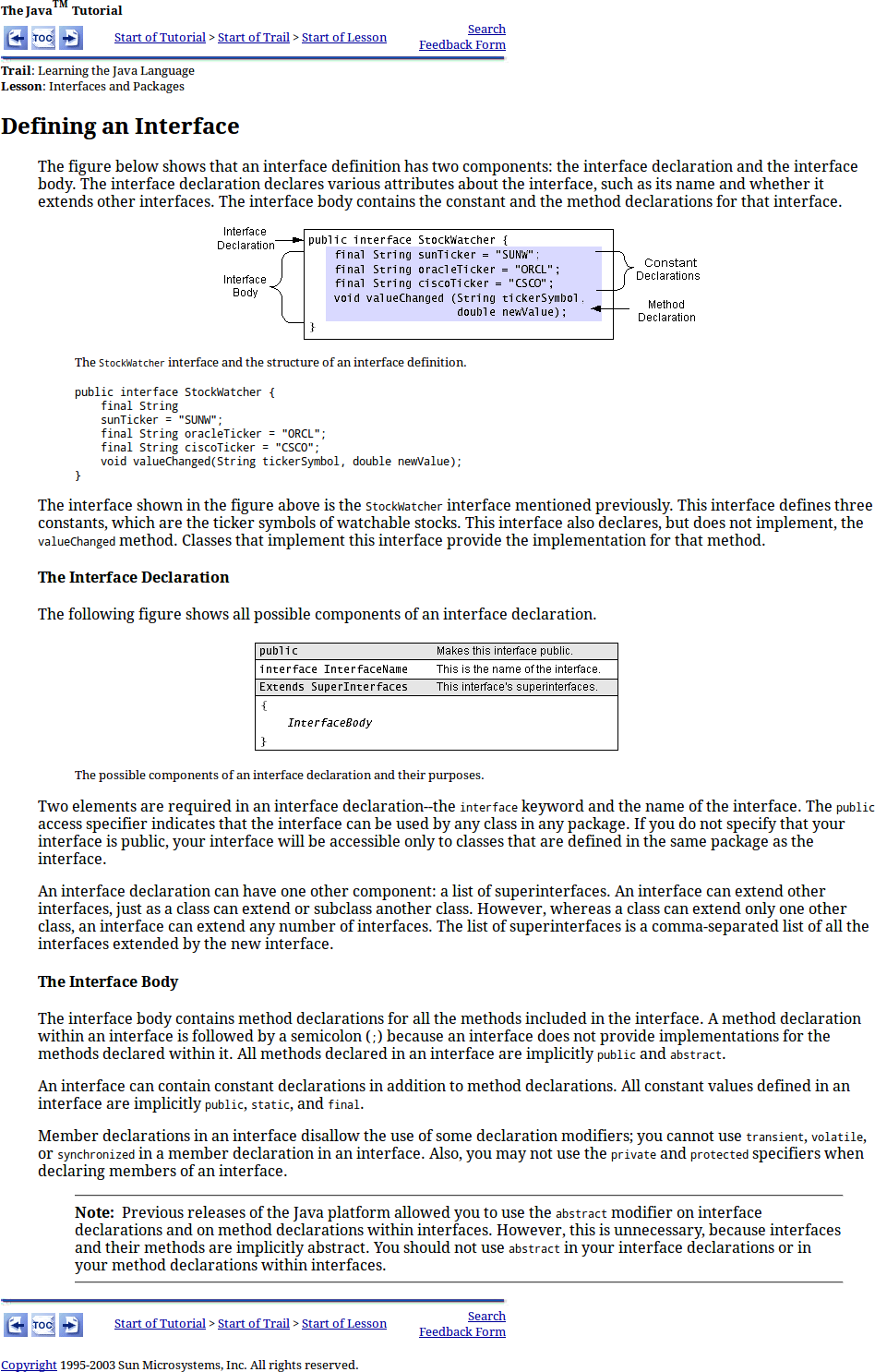
The official Java tutorial thru its update history has changed its stance about what's a interface. The following are verbatim quotes:
before :
Definition: An interface is a named collection of method definitions (without implementations). An interface can also declare constants.
sometimes after :
Definition: An interface is a device that unrelated objects -- objects that are not related by class hierarchy -- can use to interact with each other. An object can implement multiple interfaces.
Complexer and complexer. Note its use of the word “device”.